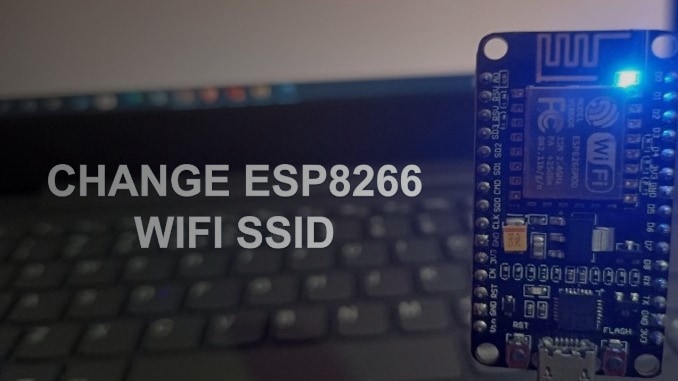
This tutorial guides you to build an ESP8266 Web Server that changes the ESP8266 wifi user and password without uploading the code again. This ESP8266 Web page can be accessed with any device with a browser using your local network.
Table of Contents
Installing Libraries
To build the ESP8266 Web Server you need to install the following libraries. You can click the following links to download the libraries.
- ESP8266WebServer
- ESP8266HTTPClient
- ArduinoJson
- FS(SPIFFS)
- Click here to download the ESP8266WebServer, ESP8266HTTPClient, ArduinoJson, and SPIFFS libraries. You should have a .zip folder in your Downloads folder.
- You can go to Sketch > Include Library > Add. ZIP library and select the library you’ve just downloaded.
Installing the ESP8266 Filesystem Uploader
- Click here to download the ESP8266FS-0.2.0.zip
- Unzip the file
- Drop the ‘esp8266fs.jar’ file into the Arduino tool directory;
Arduino code for Change ESP8266 WiFi credentials
How does this code work? follow the below steps;
- On the first ESP8266 boot, it is set up in station mode and Reads the SSID and password are pre-saved on the JSON file.
- After establishing a connection with the Access point, you can go to the default IP address 192.168.4.1 to open a web page that allows the user to configure your SSID and password.
- Once a new SSID and password are set, the ESP restarts and tries to connect with the new SSID and password.
Testing the Arduino code
- First, you can upload the code,
- Click the ESP8266 ‘Sketch data upload’, Inside that folder, create a new folder called data.
#include <ESP8266WiFi.h> #include <ESP8266HTTPClient.h> #include <ESP8266WebServer.h> #include <ArduinoJson.h> #include "FS.h" //Variables int i = 0; int statusCode; String st; String content; //Function Decalration void launchWeb(void); void setupAP(void); bool saveConfig(void); bool loadConfig(void); //Establishing Local server at port 80 whenever required ESP8266WebServer server(80); char wifi_ssid[10]; char wifi_password[15]; bool loadConfig() { File configFile = SPIFFS.open("/config.json", "r"); if(!configFile) { Serial.println("Failed to open config file:"); return false; } size_t size = configFile.size(); Serial.println("size "); Serial.println(size); if(size > 256){ Serial.println("Config file size is to large"); return false; } //Allocate a buffer to store contents of the file. std::unique_ptr<char[]> buf(new char[size]); configFile.readBytes(buf.get(), size); Serial.println(buf.get()); StaticJsonBuffer<256> jsonBuffer; JsonObject& json = jsonBuffer.parseObject(buf.get()); if(!json.success()){ Serial.println("Failed to parse config file"); return false; } const char* json_ssid = json["json_ssid"]; strncpy(wifi_ssid, json_ssid, 10); Serial.print("ssid Loaded: "); Serial.println(wifi_ssid); const char* json_paswd = json["json_paswd"]; strncpy(wifi_password, json_paswd, 15); Serial.print("paswd Loaded: "); Serial.println(wifi_password); return true; } bool saveConfig() { StaticJsonBuffer<256> jsonBuffer; JsonObject& json = jsonBuffer.createObject(); json["json_ssid"] = wifi_ssid; json["json_paswd"] = wifi_password; File configFile = SPIFFS.open("/config.json", "w"); if (!configFile) { Serial.println("Failed to open config file for writing"); return false; } json.printTo(configFile); return true; } void setup() { Serial.begin(9600); //Initialising if(DEBUG)Serial Monitor Serial.println(); Serial.println("Monting FS..."); if(!SPIFFS.begin()){ Serial.println("Failed to mount file system"); return; } loadConfig(); saveConfig(); pinMode(LED_BUILTIN, OUTPUT); Serial.print("\n"); Serial.println("Turning the HotSpot On"); launchWeb(); setupAP();// Setup HotSpot Serial.println(); Serial.println("Waiting..."); while ((WiFi.status() != WL_CONNECTED)) { delay(100); server.handleClient(); } } void loop() { } void launchWeb() { Serial.println(""); Serial.print("SoftAP IP: "); Serial.println(WiFi.softAPIP()); createWebServer(); server.begin(); Serial.println("Server started"); } void setupAP(void) { WiFi.mode(WIFI_STA); delay(100); int n = WiFi.scanNetworks(); Serial.print("\n"); Serial.println("scan done"); if (n == 0) Serial.println("no networks found"); else { Serial.print(n); Serial.println(" networks found"); for (int i = 0; i < n; ++i) { // Print SSID and RSSI for each network found Serial.print(i + 1); Serial.print(": "); Serial.print(WiFi.SSID(i)); Serial.print(" ("); Serial.print(WiFi.RSSI(i)); Serial.print(")"); Serial.println((WiFi.encryptionType(i) == ENC_TYPE_NONE) ? " " : "*"); delay(10); } } Serial.println(""); st = "<ol>"; for (int i = 0; i < n; ++i) { // Print SSID and RSSI for each network found st += "<li>"; st += WiFi.SSID(i); st += " ("; st += WiFi.RSSI(i); st += ")"; st += (WiFi.encryptionType(i) == ENC_TYPE_NONE) ? " " : "*"; st += "</li>"; } st += "</ol>"; delay(100); WiFi.softAP(wifi_ssid, wifi_password); launchWeb(); } void createWebServer() { { server.on("/", []() { IPAddress ip = WiFi.softAPIP(); String ipStr = String(ip[0]) + '.' + String(ip[1]) + '.' + String(ip[2]) + '.' + String(ip[3]); content = "<!DOCTYPE HTML>\r\n<html>ESP8266 Web Server"; content += "<form action=\"/scan\" method=\"POST\"><input type=\"submit\" value=\"scan\"></form>"; content += ipStr; content += "<p>"; content += st; content += "</p><form method='get' action='setting'><label>SSID: </label><input name='ssid' length=10><input name='pass' length=15><input type='submit'></form>"; content += "</html>"; server.send(200, "text/html", content); }); server.on("/scan", []() { //setupAP(); IPAddress ip = WiFi.softAPIP(); String ipStr = String(ip[0]) + '.' + String(ip[1]) + '.' + String(ip[2]) + '.' + String(ip[3]); content = "<!DOCTYPE HTML>\r\n<html>go back"; server.send(200, "text/html", content); }); server.on("/setting", []() { String qsid = server.arg("ssid"); String qpass = server.arg("pass"); int sid_str_len = qsid.length() + 1; char webssid[sid_str_len]; qsid.toCharArray(webssid, sid_str_len); int pass_str_len = qpass.length() + 1; char webpass[pass_str_len]; qpass.toCharArray(webpass, pass_str_len); if ((qsid.length() > 0) && (qpass.length() >= 8) && (qpass.length() <=15)) { strncpy(wifi_ssid, webssid, 10); Serial.print("writing eeprom ssid:"); Serial.println(wifi_ssid); strncpy(wifi_password, webpass, 15); Serial.print("writing eeprom pass:"); Serial.println(wifi_password); Serial.print("\n"); saveConfig(); content = "{\"Success\":\"saved to eeprom... reset to boot into new wifi\"}"; statusCode = 200; ESP.restart(); } else { content = "{\"Error\":\"404 not found\"}"; content += "{\"password length must be of 8-15\"}"; statusCode = 404; Serial.println("Sending 404"); Serial.println("password length must be of 8-15"); } server.sendHeader("Access-Control-Allow-Origin", "*"); server.send(statusCode, "application/json", content); }); } }
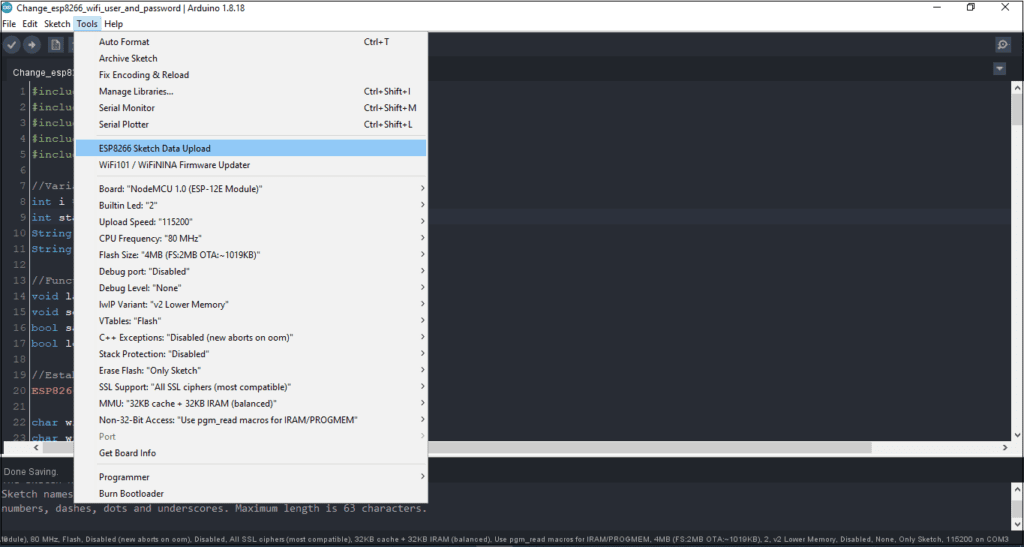
- Change the flash size,
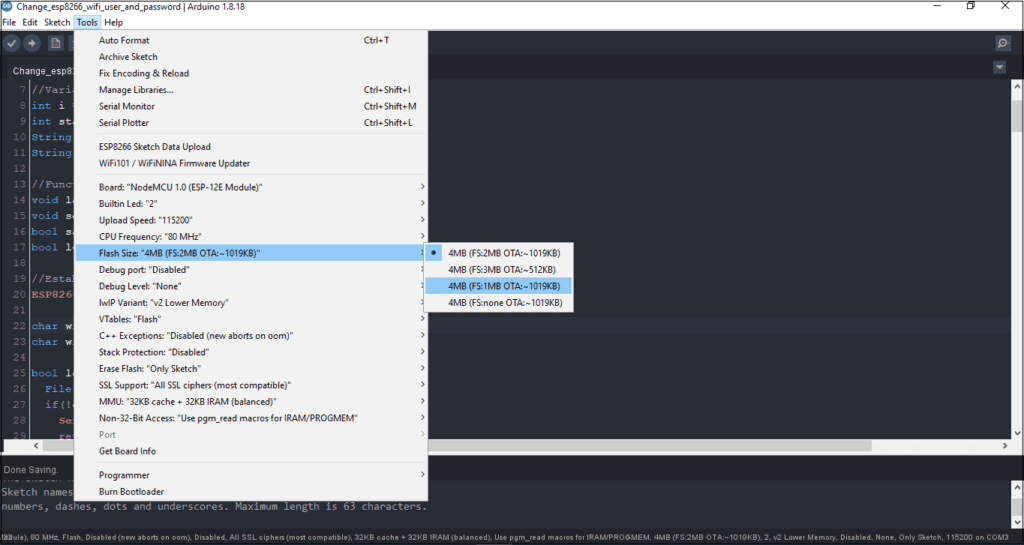
- Then upload the JSON file by clicking the ESP8266 ‘Sketch data upload’.
- Open the Serial Monitor at a baud rate of 9600 and press the ESP8266 RESET button.
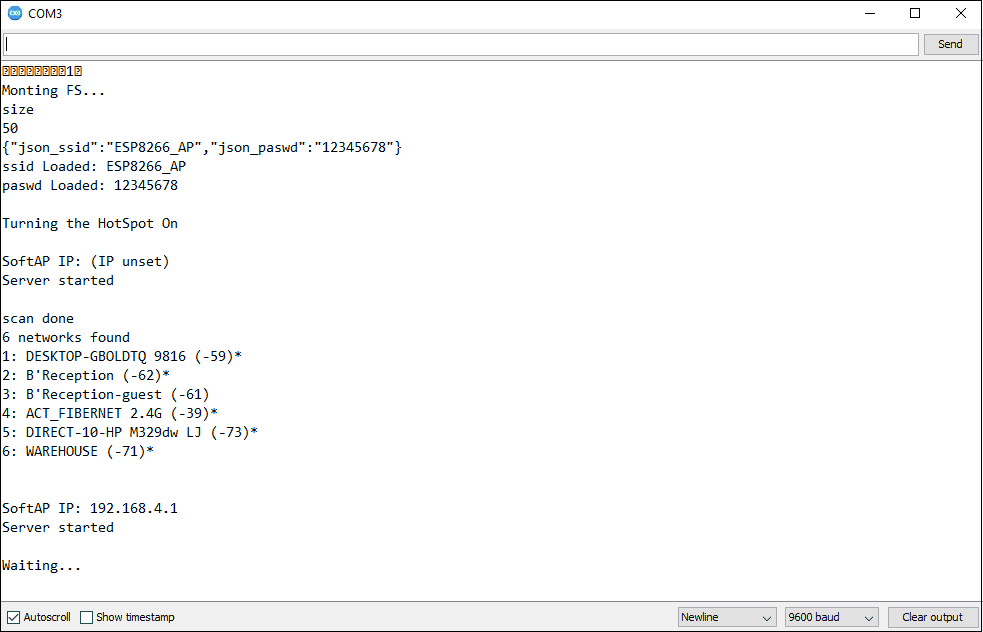
- Copy that IP address, because you need it to access the ESP8266 Web Server.
Connect to ESP8266_AP Network
Insert your network credentials, so that the ESP8266 can connect to your local wifi network.
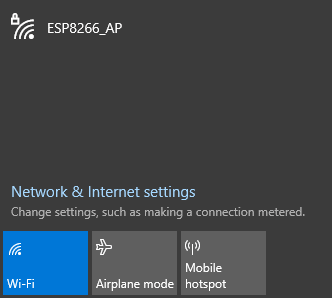
Accessing the ESP8266 Web Server page
Type the ESP8266 IP address, and you’ll see the following page.
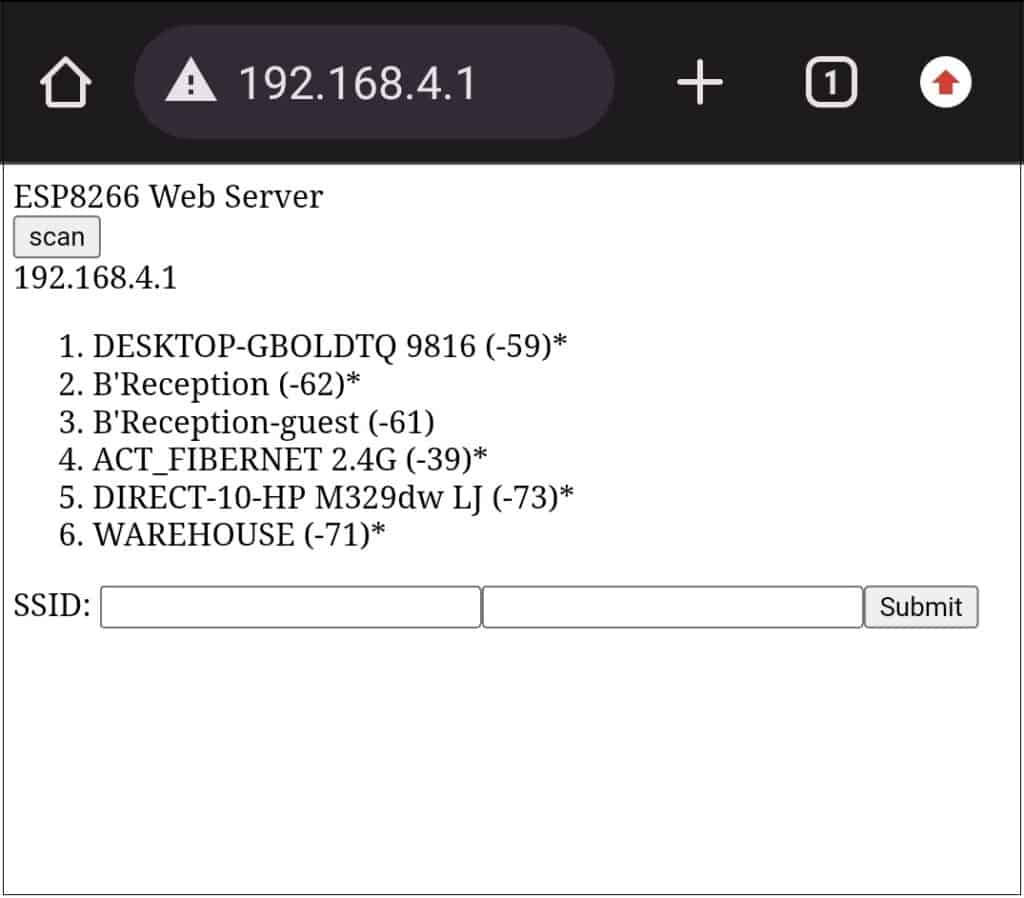
Also Read: ESP8266 Led Control using Web Server
Please convert to json 6 and littefs