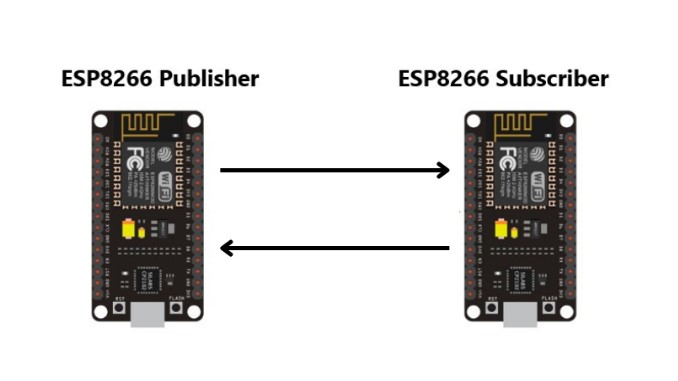
In this post, we’re going to show you how to control LED and display outputs from the ESP8266 on Node-RED. The Node-RED software is running on a local computer and the communication between the ESP8266 and the Node-RED with the MQTT communication protocol.
Project Overview
The following figure shows an overview of what we’re going to do in this project:
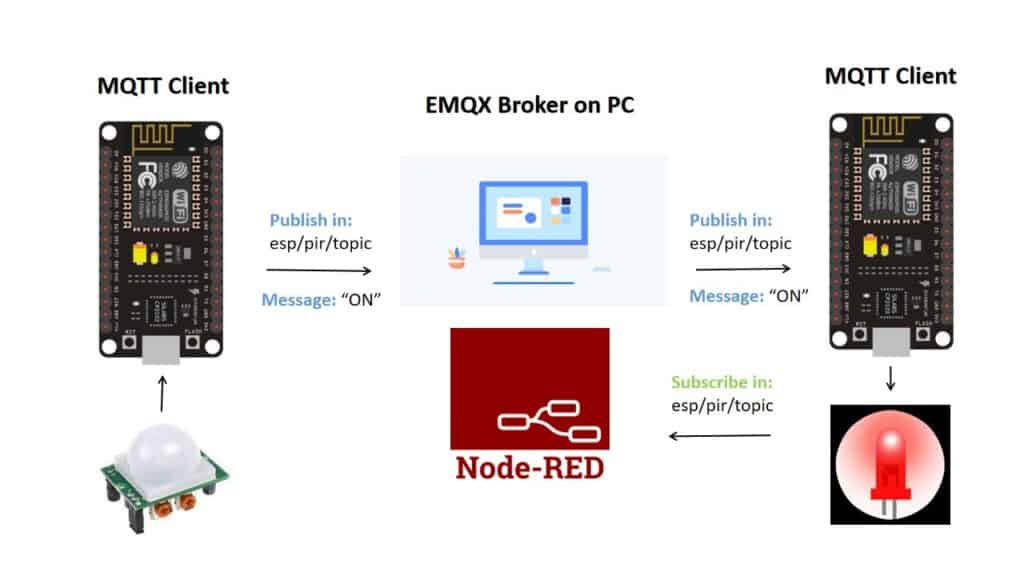
Parts Required
For this tutorial, you need the following parts:
- Two ESP8266 Development boards
- PIR Motion Sensor
- Jumper Wires
- Breadboard
Node-RED and Node-RED Dashboard
You need to have Node-RED and Node-RED Dashboard installed on your local computer.
So, We’ll cover how to install Node-RED Dashboard on your local computer.
What is Node-RED Dashboard?
Node-RED Dashboard is a module that provides a set of nodes in Node-RED to quickly create a live data dashboard. For example, it provides nodes to quickly create a user interface with buttons, sliders, charts, gauges, etc.
To learn more about Node-RED Dashboard you can check the following links:
Node-RED site: http://flows.nodered.org/node/node-red-dashboard
GitHub: https://github.com/node-red/node-red-dashboard
MQTT Protocol
In this tutorial, we’re going to establish a communication between a local computer running the Node-RED software and an ESP8266 using MQTT.
MQTT stands for MQ Telemetry Transport and it is a nice lightweight publish and subscribe system where you can publish and receive messages as a client. It is a simple messaging protocol, designed for constrained devices and with low bandwidth. So, it’s the perfect solution for Internet of Things applications.
If you want to learn more about MQTT, then see the blog post below:
- Introduction of MQTT
- Features of MQTT
- MQTT Publish-Subscribe and Unsubscribe
- MQTT Client-Broker Connection
- MQTT Topics Explained
- Quality of Service Level in MQTT
- Persistence Session & Message Queueing in MQTT
- Retained Messages in MQTT
- Last Will and Testament Feature
- MQTT Keep alive and client takeover
Installing Node-RED and EMQX Broker
Install Nodejs and Node-red
STEP 1
Install Node.js
STEP 2
EMQX MQTT Broker
- Download zip from here –> https://www.emqx.com/en/downloads/broker?osType=Linux
- Unzip the file unzip emqx-4.4.1-otp23.0-windows-amd64.zip
- Start emqx .\bin\emqx start
- Check if emqx is running .\bin\emqx_ctl status .\bin\emqx ping
- Stop emqx .\bin\emqx stop
STEP 3
Node-RED
Windows CMD:
- node –version && npm –version
- npm install -g –unsafe-perm node-red
- node-red
Installing Libraries
Installing the PubSubClient Library
- Click here to download the PubSubClient Library. You should have a .zip folder in your Downloads folder.
- You can go to Sketch > Include Library > Add. ZIP library and select the library you’ve just downloaded.
Testing an MQTT communication with Node-RED
In this section, we’re going to test an MQTT communication using the Node-RED nodes.
Creating the Flow
Drag the following nodes to the flow – see figure below:
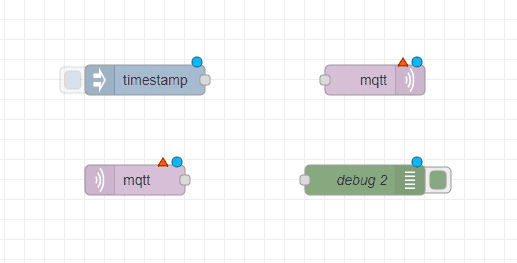
Node-RED and the MQTT broker need to be connected. To connect the MQTT broker to Node-Red, double-click the MQTT output node. A new window pops up – as shown in the figure below.
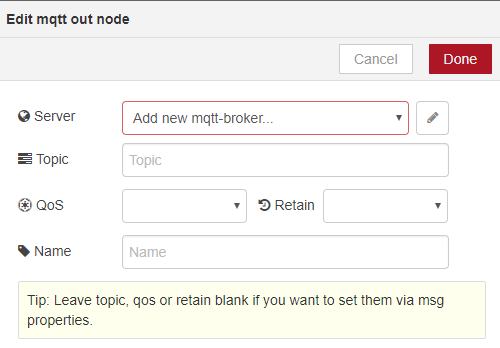
- Click the Add new MQTT-broker option
- Type localhost in the server field
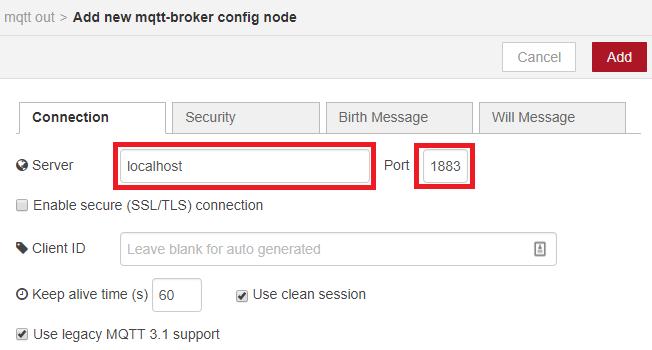
- All the other settings are configured properly by default.
- Press Add and the MQTT output node automatically connects to your broker.
Arduino Sketch for ESP8266 Publisher
#include <ESP8266WiFi.h> #include <PubSubClient.h> int inputPin = 2; int pirState = LOW; int val = 0; const int redledPin = 12; const char* ssid = "YOUR_SSID"; const char* password = "YOUR_PASSWORD"; const char* mqtt_server = "YOUR IP"; WiFiClient espClient; PubSubClient client(espClient); void setup_wifi() { delay(10); Serial.println(); Serial.print("Connecting to "); Serial.println(ssid); WiFi.begin(ssid, password); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } Serial.println(""); Serial.print("WiFi connected - ESP IP address: "); Serial.println(WiFi.localIP()); } void reconnect() { while (!client.connected()) { Serial.print("Attempting MQTT connection..."); if (client.connect("ESP8266Client")) { Serial.println("connected"); client.subscribe("room/lamp"); } else { Serial.print("failed, rc="); Serial.print(client.state()); Serial.println(" try again in 5 seconds"); delay(5000); } } } void setup() { Serial.begin(115200); pinMode(inputPin, INPUT); // declare sensor as input pinMode(redledPin, OUTPUT); setup_wifi(); client.setServer(mqtt_server, 21080); } void loop() { if (!client.connected()) { reconnect(); } if(!client.loop()) client.connect("ESP8266Client"); val = digitalRead(inputPin); if (val == HIGH) { if (pirState == LOW) { Serial.println("Motion detected!"); digitalWrite(redledPin, HIGH); pirState = HIGH; client.publish("esp/pir/topic", "ON"); } } else { if (pirState == HIGH) { Serial.println("Motion ended!"); digitalWrite(redledPin, LOW); pirState = LOW; client.publish("esp/pir/topic", "OFF"); } } }
Arduino Sketch for ESP8266 Subscriber
#include <ESP8266WiFi.h> #include <PubSubClient.h> const char* ssid = "YOUR_SSID"; const char* password = "YOUR_PASSWORD"; const char *MQTT_HOST = "YOUR _IP"; const int MQTT_PORT = 1883; const char *MQTT_CLIENT_ID = "ESP8266 NodeMCU"; const char *MQTT_USER = "user"; const char *MQTT_PASSWORD = "password"; const char *TOPIC = "esp/pir/topic"; const int ledPin = 2; WiFiClient client; PubSubClient mqttClient(client); void callback(char* topic, byte* payload, unsigned int length) { String message; for (int i = 0; i < length; i++) { message += (char)payload[i]; } if (String(topic) == "esp/pir/topic") { if(message == "ON"){ Serial.println("on"); digitalWrite(ledPin, HIGH); } else if(message == "OFF"){ Serial.println("off"); digitalWrite(ledPin, LOW); } } } void setup() { Serial.begin(115200); pinMode(ledPin, OUTPUT); WiFi.begin(ssid, password); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } Serial.println("Connected to Wi-Fi"); mqttClient.setServer(MQTT_HOST, MQTT_PORT); mqttClient.setCallback(callback); while (!client.connected()) { if (mqttClient.connect(MQTT_CLIENT_ID, MQTT_USER, MQTT_PASSWORD)) { Serial.println("Connected to MQTT broker"); } else { delay(500); Serial.print("."); } } mqttClient.subscribe(TOPIC); } void loop() { mqttClient.loop(); }
Also Read: IoT Weather Station Using Sense-Hat
Be the first to comment