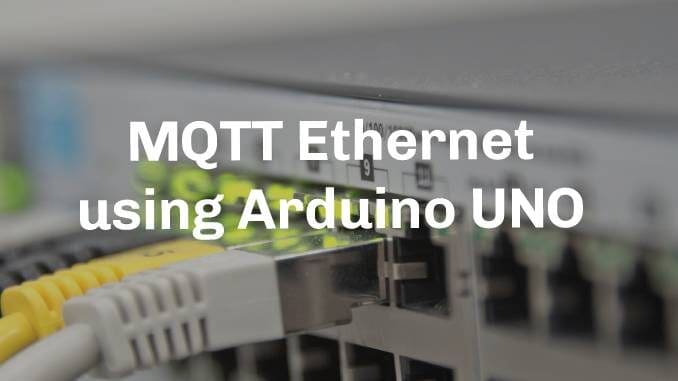
In this post we’re going to show you example of publish and subscribe with MQTT Ethernet using Arduino UNO board testing with NodeRed.
The communication between NodeRed and Arduino UNO with the help of MQTT communication protocol.
Schematic Overview
The following figure shows wire connection and overview of this project,
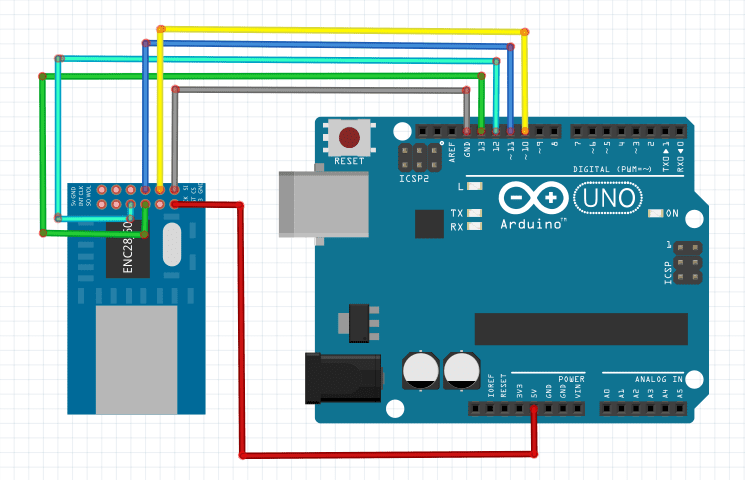
Parts Required
For this tutorial, you need the following parts:
- Arduino UNO Development board
- Ethernet Module (ENC80J60)
- Jumper Wires
- Breadboard
If you want to learn more about MQTT, then see the blog post below:
- Introduction of MQTT
- Features of MQTT
- MQTT Publish-Subscribe and Unsubscribe
- MQTT Client-Broker Connection
- MQTT Topics Explained
- Quality of Service Level in MQTT
- Persistence Session & Message Queueing in MQTT
- Retained Messages in MQTT
- Last Will and Testament Feature
- MQTT Keep alive and client takeover
Installing Libraries
Installing the PubSubClient Library
- Click here to download the PubSubClient Library. You should have a .zip folder in your Downloads folder.
- You can go to Sketch > Include Library > Add. ZIP library and select the library you’ve just downloaded.
Installing the EthernetENC Library
- Click here to download the EthernetENC Library. You should have a .zip folder in your Downloads folder.
- You can go to Sketch > Include Library > Add. ZIP library and select the library you’ve just downloaded.
MQTT Broker Connection
Node-RED and the MQTT broker need to be connected. Drag the following nodes to the flow.
To connect the MQTT broker to Node-Red, double-click the node. A new window pops up – as shown in the figure below.
mqtt out to publish the message to arduino UNO
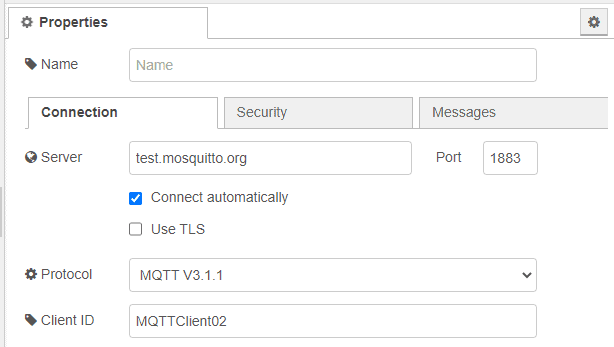
- Set topic UnoInTopic in the topic field and set QoS to 0
- Click the Add new MQTT-broker option
- Type test.mosquitto.org in the server field and set mqtt port 1883 in the port field
mqtt in to see the output of UnoInTopic
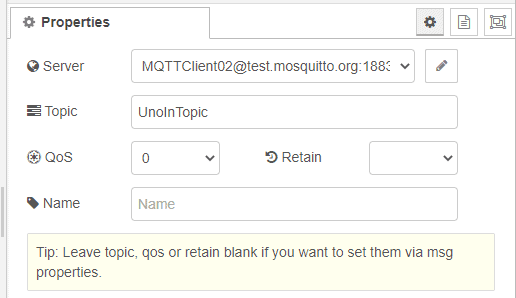
- Set topic UnoInTopic in the topic field and set QoS to 0
- Click the Add new MQTT-broker option same as mqtt out
- Type test.mosquitto.org in the server field and set mqtt port 1883 in the port field
mqtt in to see the message are received from arduino UNO
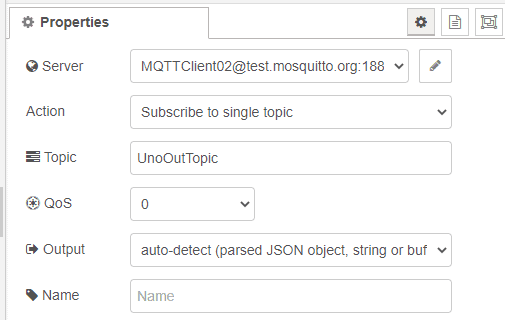
- Set topic UnoOutTopic in the topic field and set QoS to 0
- Click the Add new MQTT-broker option same as mqtt out
- Type test.mosquitto.org in the server field and set mqtt port 1883 in the port field
Arduino Code for MQTT Client
// Basic MQTT example #include <PubSubClient.h> #include <EthernetENC.h> // Update these with values suitable for your network. uint8_t mac[6] = {0xDE, 0xED, 0xBA, 0xFE, 0xFE, 0xED}; IPAddress ip(192, 168, 10, 143); //IPAddress server(192, 168, 9, 137); EthernetClient ethClient; PubSubClient mqttClient(ethClient); void callback(char* topic, byte* payload, unsigned int length) { Serial.print("Message arrived ["); Serial.print(topic); Serial.print("] "); for (int i=0;i<length;i++) { Serial.print((char)payload[i]); } Serial.println(); } void reconnect() { // Loop until we're reconnected while (!mqttClient.connected()) { Serial.print("Attempting MQTT connection..."); delay(1000); // Attempt to connect if (mqttClient.connect("MQTTClient01")) { Serial.println("connected"); // Once connected, publish an announcement... mqttClient.publish("UnoOutTopic","hello world from UNO!"); // ... and resubscribe mqttClient.subscribe("UnoInTopic"); } else { Serial.print("failed, rc="); Serial.print(mqttClient.state()); Serial.println(" try again in 5 seconds"); // Wait 5 seconds before retrying delay(5000); } } } void setup() { Serial.begin(115200); Ethernet.begin(mac, ip); // Ethernet.begin(mac); // Allow the hardware to sort itself out delay(1000); Serial.print("localIP: "); Serial.println(Ethernet.localIP()); Serial.print("subnetMask: "); Serial.println(Ethernet.subnetMask()); Serial.print("gatewayIP: "); Serial.println(Ethernet.gatewayIP()); Serial.print("dnsServerIP: "); Serial.println(Ethernet.dnsServerIP()); // connect to mqtt broker mqttClient.setServer("test.mosquitto.org", 1883); // mqttClient.setServer(server, 1883); mqttClient.setCallback(callback); delay(5000); } void loop() { if (!mqttClient.connected()) { reconnect(); } mqttClient.loop(); }
Testing an MQTT Client with Serial Monitor
Now, you can upload the code, open the serial monitor at a baud rate of 115200 and press the Arduino UNO RESET button.
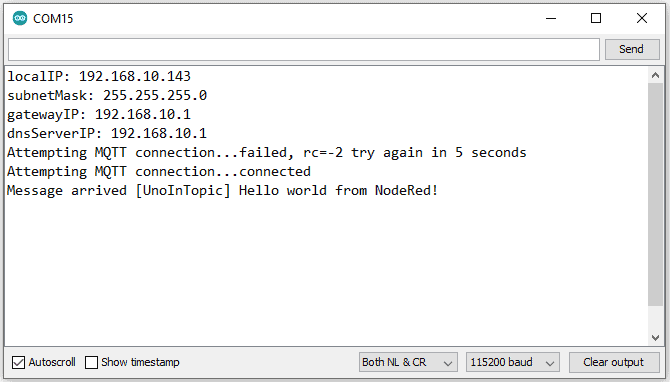
Testing an MQTT Client with Node-RED
In this section, we’re going to test an MQTT communication using the Node-RED nodes.
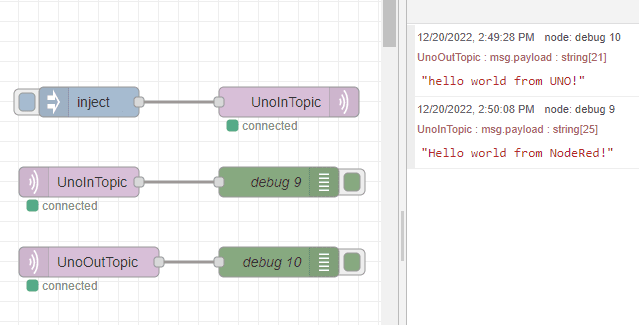
Github Link: download the project files
Be the first to comment